Difference between revisions of "W7003 The Basics"
Line 1: | Line 1: | ||
== First Steps == | == First Steps == | ||
=== Prepare a Directory === | |||
{{ConsoleLines| | |||
{{Lime|john-williams@codermerlin}}:{{Cyan|~/}}$ cd<br/> | |||
{{Lime|john-williams@codermerlin}}:{{Cyan|~/}}$ mkdir AP-Practice<br/> | |||
{{Lime|john-williams@codermerlin}}:{{Cyan|~/}}$ cd AP-Practice<br/> | |||
* | {{Lime|john-williams@codermerlin}}:{{Cyan|~/AP-Practice}}$ mkdir HelloWorld<br/> | ||
* An executable must have an entry point which is a '''public''', '''static''' function named ''main'', accepting a single argument, an array of strings. | {{Lime|john-williams@codermerlin}}:{{Cyan|~/AP-Practice}}$ cd HelloWorld<br/> | ||
{{Lime|john-williams@codermerlin}}:{{Cyan|~/AP-Practice/HelloWorld}}$ <br/> | |||
}} | |||
=== Create a New File === | |||
{{ConsoleLines| | |||
{{Lime|john-williams@codermerlin}}:{{Cyan|~/AP-Practice/HelloWorld}}$ emacs HelloWorld.java<br/> | |||
}} | |||
Enter the following text: | |||
<syntaxhighlight lang="java"> | |||
public class HelloWorld { | |||
public static void main(String[] args) { | |||
System.out.println("Hello, World!"); | |||
} | |||
} | |||
</syntaxhighlight> | |||
{{Hint| | |||
* '''Names matter''' - The file name MUST match the name of the public class | |||
* An executable must have an ''entry point'' which is a '''public''', '''static''' function named ''main'', accepting a single argument, an array of strings. | |||
}} | |||
=== Compilation === | |||
A Java program can be compiled with the '''javac''' command. Note that the argument to the command is the file to be compiled, including the ".java" suffix. This will produce a file with the ".class" suffix. | |||
{{ConsoleLines| | |||
{{Lime|john-williams@codermerlin}}:{{Cyan|~/AP-Practice/HelloWorld}}$ javac HelloWorld.java<br/> | |||
}} | |||
=== Execution === | |||
A Java program may be executed with the '''java''' command. Note that the argument to the command is the file to be executed, excluding the ".class" suffix. | |||
{{ConsoleLines| | |||
{{Lime|john-williams@codermerlin}}:{{Cyan|~/AP-Practice/HelloWorld}}$ java HelloWorld<br/> | |||
}} | |||
== Short Cuts == | == Short Cuts == |
Revision as of 22:32, 27 November 2019
First Steps[edit]
Prepare a Directory[edit]
john-williams@codermerlin:~/$ cd
john-williams@codermerlin:~/$ mkdir AP-Practice
john-williams@codermerlin:~/$ cd AP-Practice
john-williams@codermerlin:~/AP-Practice$ mkdir HelloWorld
john-williams@codermerlin:~/AP-Practice$ cd HelloWorld
john-williams@codermerlin:~/AP-Practice/HelloWorld$
Create a New File[edit]
john-williams@codermerlin:~/AP-Practice/HelloWorld$ emacs HelloWorld.java
Enter the following text:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
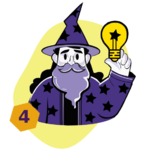
- Names matter - The file name MUST match the name of the public class
- An executable must have an entry point which is a public, static function named main, accepting a single argument, an array of strings.
Compilation[edit]
A Java program can be compiled with the javac command. Note that the argument to the command is the file to be compiled, including the ".java" suffix. This will produce a file with the ".class" suffix.
john-williams@codermerlin:~/AP-Practice/HelloWorld$ javac HelloWorld.java
Execution[edit]
A Java program may be executed with the java command. Note that the argument to the command is the file to be executed, excluding the ".class" suffix.
john-williams@codermerlin:~/AP-Practice/HelloWorld$ java HelloWorld
Short Cuts[edit]
- Compilation and Execution can be combined
- All of this can occur within emacs
- Emacs shortcut and up arrow
- Define keyboard macro with f3 and f4, execute with f4
- CTRL-X, CTRL-S, ALT-SHIFT-&, Up-Arrow, ENTER
Semicolons[edit]
Semicolons are required at the end of each statement
Static Typing[edit]
- Java is statically typed
- Variables must be declared before they can be used, including the type
- Types define the possible values of the variable and the operations that may be performed upon it
Primitive Types[edit]
NOTE: All primitive types begin with a lowercase letter
- byte (8-bit)
- short (16-bit)
- int (32-bit)
- long (64-bit)
- boolean
- char (16-bit unicode)
- float
- double
Common Errors[edit]
int gpa;
gpa = 3.75;
float f = 10.5;
byte b = -128;
b -= 1;
Implicit Casting[edit]
- Magnitude of numeric types is preserved (precision may be lost)
Explicit Casting[edit]
- Required when magnitude of numeric type may not be preserved
Division by Zero[edit]
- Compare and contrast integer vs floating point
New[edit]
The new keyword is not used for primitive types
Primitive numeric types[edit]
- Primitive numeric types overflow and underflow silently