Difference between revisions of "W1523 Paddle Paddle"
Jeff-strong (talk | contribs) m (Editorial review and minor corrections) |
|||
(17 intermediate revisions by 5 users not shown) | |||
Line 1: | Line 1: | ||
[[File:Boy and girl play ping-pong, circa 1950 (4710047377).jpg|thumb| | [[File:Boy and girl play ping-pong, circa 1950 (4710047377).jpg|thumb|A boy and girl play ping-pong, circa 1950]] | ||
== Prerequisites == | == Prerequisites == | ||
* [[W1522 Ping Then Pong]] | * [[W1522 Ping Then Pong]] | ||
Line 6: | Line 6: | ||
== Background == | == Background == | ||
As we learned in the previous lab, the {{SwiftIdentifier|render}} event handler is invoked by the system periodically to refresh the canvas. | As we learned in the previous lab, the {{SwiftIdentifier|render}} event handler is invoked by the system periodically to refresh the canvas. We'll take advantage of this behavior to draw two "paddles"—one on the left and one on the right. We'll also learn how to handle keyboard input. | ||
== Experiment == | == Experiment == | ||
=== Getting Started === | === Getting Started === | ||
Continue from the previous project; we'll be editing all of our files there. | Continue from the previous project; we'll be editing all of our files there. Enter into the the project's Sources directory. | ||
{{ConsoleLine|john-williams@codermerlin:|cd ~/Experiences/W1521/Sources/ScenesShell/}} | {{ConsoleLine|john-williams@codermerlin:|cd ~/Experiences/W1521/Sources/ScenesShell/}} | ||
=== First Steps === | === First Steps === | ||
Let's start by adding a new file to our project, | Let's start by adding a new file to our project, {{Pathname|Paddle.swift}}. Open the file for editing. | ||
=== Add Constructor and Required Methods === | === Add Constructor and Required Methods === | ||
Our paddle will be a rectangle. | Our paddle will be a rectangle. Feel free to use any colors for stroke and fill that you deem appropriate. | ||
<syntaxhighlight lang="swift"> | <syntaxhighlight lang="swift"> | ||
import Igis | import Igis | ||
import Scenes | |||
class Paddle { | class Paddle: RenderableEntity { | ||
var rectangle : Rectangle | var rectangle: Rectangle | ||
init( | init(rect:Rect) { | ||
rectangle = Rectangle(rect:rect, fillMode:.fillAndStroke) | rectangle = Rectangle(rect:rect, fillMode:.fillAndStroke) | ||
// Using a meaningful name can be helpful for debugging | |||
super.init(name: "Paddle") | |||
} | } | ||
func | override func render(canvas:Canvas) { | ||
let strokeStyle = StrokeStyle(color:Color(.black)) | let strokeStyle = StrokeStyle(color:Color(.black)) | ||
let fillStyle = FillStyle(color:Color(.white)) | let fillStyle = FillStyle(color:Color(.white)) | ||
let lineWidth = LineWidth(width:2) | let lineWidth = LineWidth(width:2) | ||
canvas. | canvas.render(strokeStyle, fillStyle, lineWidth, rectangle) | ||
} | } | ||
func move(to:Point) { | func move(to point:Point) { | ||
rectangle.rect.topLeft = | rectangle.rect.topLeft = point | ||
} | } | ||
Line 48: | Line 47: | ||
</syntaxhighlight> | </syntaxhighlight> | ||
=== Add a Left Paddle to Our | === Add a Left Paddle to Our InteractionLayer === | ||
We'll need to make the following changes to our | We'll need to make the following changes to our '''InteractionLayer''' class: | ||
# Add a | |||
# | # import the {{SwiftIdentifier|Igis}} library | ||
# Set the initial coordinates of | # Add a {{SwiftIdentifier|paddleLeft}} property and initialize {{SwiftIdentifier|paddleLeft}} | ||
# Insert the {{SwiftIdentifier|paddleLeft}} into the {{SwiftIdentifier|InteractionLayer}} | |||
# Set the initial coordinates of {{SwiftIdentifier|paddleLeft}} in a new, override func {{SwiftIdentifier|preSetup}} | |||
<syntaxhighlight lang="swift" highlight="1"> | |||
import Igis | |||
</syntaxhighlight> | |||
<syntaxhighlight lang="swift" highlight="2"> | <syntaxhighlight lang="swift" highlight="2"> | ||
let ball | let ball = Ball() | ||
let paddleLeft : | let paddleLeft = Paddle(rect:Rect(size:Size(width:10, height:100))) | ||
</syntaxhighlight> | </syntaxhighlight> | ||
<syntaxhighlight lang="swift" highlight=" | <syntaxhighlight lang="swift" highlight="8"> | ||
init() { | |||
// Using a meaningful name can be helpful for debugging | |||
super.init(name:"Interaction") | |||
insert(entity: ball, at: .front) | |||
ball.changeVelocity(velocityX: 3, velocityY: 5) | |||
insert(entity: paddleLeft, at: .front) | |||
} | } | ||
</syntaxhighlight> | </syntaxhighlight> | ||
<syntaxhighlight lang="swift" highlight="7"> | <syntaxhighlight lang="swift" highlight="7"> | ||
func | override func preSetup(canvasSize: Size, canvas: Canvas) { | ||
paddleLeft.move(to:Point(x: 10, y: canvasSize.center.y)) | |||
} | |||
</syntaxhighlight> | |||
{{RunProgram|Run the program and view in a browser before continuing. Ensure that the application behaves as expected.}} | |||
=== Add a Right Paddle to Our InteractionLayer === | |||
Using what you've just learned, add a right paddle to the code. | |||
{{Observe|Section 1| | |||
Is it necessary to define another '''class''' to add another paddle? Why or why not?}} | |||
{{RunProgram|Run the program and view in a browser before continuing. Ensure that the application behaves as expected and that a paddle is on the left of the screen and another is on the right.}} | |||
=== Add an Event Handler to Process Key Down Events === | |||
Add the following method to our {{SwiftIdentifier|'''InteractionLayer'''}} class: | |||
<syntaxhighlight lang="swift"> | |||
func onKeyDown(key:String, code:String, ctrlKey:Bool, shiftKey:Bool, altKey:Bool, metaKey:Bool) { | |||
} | |||
</syntaxhighlight> | |||
Enable the onKeyDown handler by making the following changes: | |||
<syntaxhighlight lang="swift" highlight="1,8,11-13"> | |||
class InteractionLayer : Layer, KeyDownHandler | |||
... | |||
override func preSetup(canvasSize: Size, canvas: Canvas) { | |||
paddleLeft.move(to:Point(x: 10, y: canvasSize.center.y)) | |||
dispatcher.registerKeyDownHandler(handler: self) | |||
} | |||
override func postTeardown() { | |||
dispatcher.unregisterKeyDownHandler(handler: self) | |||
override func | |||
} | } | ||
</syntaxhighlight> | </syntaxhighlight> | ||
{{Hint| | |||
Thoroughly review the [https://github.com/TheCoderMerlin/ScenesContainmentExample Scenes Containment Example]. How can '''containment''' help you provide the required functionality? | |||
}} | |||
== Exercises == | == Exercises == | ||
{{W1523-Exercises}} | |||
* {{MMMAssignment|M1523-28}} | |||
== Key Concepts == | == Key Concepts == | ||
[[Category:IGIS]] |
Latest revision as of 16:05, 25 May 2022
Prerequisites[edit]
Research[edit]
Background[edit]
As we learned in the previous lab, the render event handler is invoked by the system periodically to refresh the canvas. We'll take advantage of this behavior to draw two "paddles"—one on the left and one on the right. We'll also learn how to handle keyboard input.
Experiment[edit]
Getting Started[edit]
Continue from the previous project; we'll be editing all of our files there. Enter into the the project's Sources directory.
john-williams@codermerlin: cd ~/Experiences/W1521/Sources/ScenesShell/
First Steps[edit]
Let's start by adding a new file to our project, Paddle.swift. Open the file for editing.
Add Constructor and Required Methods[edit]
Our paddle will be a rectangle. Feel free to use any colors for stroke and fill that you deem appropriate.
import Igis
import Scenes
class Paddle: RenderableEntity {
var rectangle: Rectangle
init(rect:Rect) {
rectangle = Rectangle(rect:rect, fillMode:.fillAndStroke)
// Using a meaningful name can be helpful for debugging
super.init(name: "Paddle")
}
override func render(canvas:Canvas) {
let strokeStyle = StrokeStyle(color:Color(.black))
let fillStyle = FillStyle(color:Color(.white))
let lineWidth = LineWidth(width:2)
canvas.render(strokeStyle, fillStyle, lineWidth, rectangle)
}
func move(to point:Point) {
rectangle.rect.topLeft = point
}
}
Add a Left Paddle to Our InteractionLayer[edit]
We'll need to make the following changes to our InteractionLayer class:
- import the Igis library
- Add a paddleLeft property and initialize paddleLeft
- Insert the paddleLeft into the InteractionLayer
- Set the initial coordinates of paddleLeft in a new, override func preSetup
import Igis
let ball = Ball()
let paddleLeft = Paddle(rect:Rect(size:Size(width:10, height:100)))
init() {
// Using a meaningful name can be helpful for debugging
super.init(name:"Interaction")
insert(entity: ball, at: .front)
ball.changeVelocity(velocityX: 3, velocityY: 5)
insert(entity: paddleLeft, at: .front)
}
override func preSetup(canvasSize: Size, canvas: Canvas) {
paddleLeft.move(to:Point(x: 10, y: canvasSize.center.y))
}
![]() |
Run the program and view in a browser before continuing. Ensure that the application behaves as expected. |
Add a Right Paddle to Our InteractionLayer[edit]
Using what you've just learned, add a right paddle to the code.
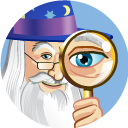
Is it necessary to define another class to add another paddle? Why or why not?
![]() |
Run the program and view in a browser before continuing. Ensure that the application behaves as expected and that a paddle is on the left of the screen and another is on the right. |
Add an Event Handler to Process Key Down Events[edit]
Add the following method to our InteractionLayer class:
func onKeyDown(key:String, code:String, ctrlKey:Bool, shiftKey:Bool, altKey:Bool, metaKey:Bool) {
}
Enable the onKeyDown handler by making the following changes:
class InteractionLayer : Layer, KeyDownHandler
...
override func preSetup(canvasSize: Size, canvas: Canvas) {
paddleLeft.move(to:Point(x: 10, y: canvasSize.center.y))
dispatcher.registerKeyDownHandler(handler: self)
}
override func postTeardown() {
dispatcher.unregisterKeyDownHandler(handler: self)
}
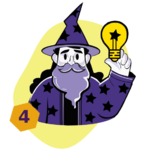
Thoroughly review the Scenes Containment Example. How can containment help you provide the required functionality?
Exercises[edit]

- Use print statements to investigate the arguments provided to the
onKeyDown
event handler - Select sensible keys to be used to move the left paddle up and down and to move the right paddle up and down
- Implement the required code to actually move the paddles in accordance with your selected keypresses
- M1523-28 Complete Merlin Mission Manager Mission M1523-28.