Difference between revisions of "W1351 Swap Function"
From Coder Merlin
m (Merlin moved page W1851 Swap Function to W1351 Swap Function without leaving a redirect: Improved navigation) |
|||
Line 1: | Line 1: | ||
[[File:Othello-Standard-Board.jpg|thumb|link=|Othello Board]] | |||
== Prerequisites == | == Prerequisites == | ||
* [[W1301 Arrays]] | |||
== Introduction == | |||
A very common need in many algorithms is swapping the values in two variables. It's also very common that these two variables are contained within an array. | |||
== Swapping == | |||
The first approach that most students take when swapping is this: | |||
<syntaxhighlight lang="swift"> | |||
let x = 5 | |||
let y = 7 | |||
x = y | |||
y = x | |||
</syntaxhighlight> | |||
Take the time to think through what you believe will occur as the above code is executed. Then, try it. | |||
{{Observe|Section 1| | |||
# What syntax errors do you observe in the above code? | |||
# What logic errors do you observe in the above code? | |||
}} | |||
The primary issue that most students encounter in this scenario is '''overwriting''' the value of one of the variables, and then assigning this overwritten value to the other value. | |||
{{Observe|Section 2| | |||
Assuming that the syntax errors in the above code segment are resolved: | |||
# What will be the value of '''x''' after the code executes? | |||
# What will be the value of '''y''' after the code executes? | |||
}} | |||
View the following video: [https://www.youtube.com/watch?v=vzCUWAxq6lU Swapping Algorithm] (YouTube) | |||
{{Observe|Section 3| | |||
# What must be done to properly swap the values of two variables? | |||
}} | |||
== Exercises == |
Revision as of 19:21, 29 March 2020
Within these castle walls be forged Mavens of Computer Science ...
— Merlin, The Coder
Prerequisites[edit]
Introduction[edit]
A very common need in many algorithms is swapping the values in two variables. It's also very common that these two variables are contained within an array.
Swapping[edit]
The first approach that most students take when swapping is this:
let x = 5
let y = 7
x = y
y = x
Take the time to think through what you believe will occur as the above code is executed. Then, try it.
Observe
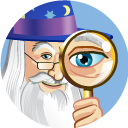
Observe, Ponder, and Journal: Section 1
- What syntax errors do you observe in the above code?
- What logic errors do you observe in the above code?
The primary issue that most students encounter in this scenario is overwriting the value of one of the variables, and then assigning this overwritten value to the other value.
Observe
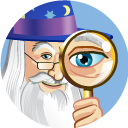
Observe, Ponder, and Journal: Section 2
Assuming that the syntax errors in the above code segment are resolved:
- What will be the value of x after the code executes?
- What will be the value of y after the code executes?
View the following video: Swapping Algorithm (YouTube)
Observe
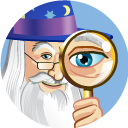
Observe, Ponder, and Journal: Section 3
- What must be done to properly swap the values of two variables?