Operating Systems
Prerequisites[edit]
Background[edit]
- Read File systems and operating systems on Wikipedia
Introduction[edit]
As the name suggests, an Operating System handles all operations of a computer system. With ever-increasing quantities of hardware in the world paired with distinct mechanisms to control each of them, it became a necessity for computer users to have a middleman program that knows how to deal with these peripherals and present them with a simple interface to interact with the computer. Hence, our good programmers created the Operating System which acts as a universal program that takes care of all the ones and zeros of a computer and its components.
Where OS Stands[edit]
Take a look at this graph showing the architecture of a computer system:
We can see that the Operating System sits somewhat in the middle, acting as the sole mediator between the humans and the computer's hardware.
Let's examine how an Operating System performs these tasks for us, shall we?
Program Execution[edit]
First off, let's observe a step-by-step explanation of how an Operating System helps a program to execute:
- Firstly, the user launches the program.
This could be a simple program double-click from inside a file explorer GUI or a direct command input from a console ordering a program to launch. - Secondly, Upon receiving this launch command the Operating System allocates a physical memory (RAM) block just for this program.
- Thirdly, the OS loads any program code currently saved in the secondary memory (e.g., HDD/SSD) onto the RAM block.
- As the final step, the Operating System instructs the CPU to run the program.
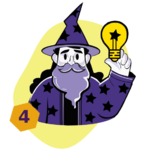

But this doesn't necessarily mean the CPU will run it, per se. CPU might run the program straightaway, or not. This is simply because of the different states a process passes through as it executes.
Let's take a look at it in the next section.Process Execution Stages[edit]
An Operating System makes a process traverse through many states:
At any given time a started process could be in any one of these states:
- new - Process is getting created.
- ready - Process is ready to start running, and awaiting instructions from the CPU.
- running - Process is running. CPU is executing instructions given by the process.
- waiting - Process is paused and waiting for an event to occur, e.g., keyboard input.
- terminated - Process has completed.
Process Scheduling[edit]
As we saw in the previous topic, a process waits for CPU instructions to move from ready state to running. It's the duty of the Operating System to analyze and determine which processes to be moved to running state and which of them to be kept on the ready. This function of the Operating System is called Process Scheduling. Operating System tries to have the CPU busy executing processes while also ensuring each program receives the minimum response time possible.
Scheduling Categories[edit]
Process scheduling can be categorized based on how the currently running process is chosen:
- Preemptive Scheduling
CPU time is divided into time slots and the processes are given a slot to switch to running state and execute. If a process couldn't complete its execution within the time slot given, it is moved to the ready state until it's called back. - Non-preemptive Scheduling
A process is kept in the running state until it finishes its execution or moved to a waiting state (e.g., waiting for I/O event completion). In the case of the latter, another process will be given the chance to execute.
Memory Management[edit]
As much as the CPU, the Operating System is responsible for managing the Physical memory (RAM) and secondary memory (e.g., HDD/SSD) of a computer. When a program is running, the Operating System loads required program data currently stored in the Secondary memory onto memory blocks of the Main memory.
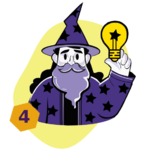
The OS keeps track of memory locations on which of them are currently allocated to a program and which of them are free. Based on the currently running processes requesting for memory, the Operating System analyzes and determines how much memory each of these processes can receive and when it can expect the memory to be allocated.
Virtual Memory Allocation[edit]
Operating Systems follow a concept called Virtual Memory allocation to avoid non-sequential blocks of memory being allocated to programs.
Let's assume a program 'foo' was started and the Operating System allocated a memory block containing of addresses 0 through 999 for this program. Later another program 'bar' was started and it was allocated addresses 1000 through 1999.
Physical Memory Address | Allocated Program |
---|---|
0-999 | foo |
1000-1999 | bar |
2000-2999 | - |
Afterwards, 'foo' was out of memory and requested for more from the Operating System. But since the sequential memory addresses were already allocated by 'bar', the OS could only grant the next memory block of addresses 2000-2999.
Physical Memory Address | Allocated Program |
---|---|
0-999 | foo |
1000-1999 | bar |
2000-2999 | foo |
3000-3999 | - |
As you can see, this introduced a discontinuous order for the physical addresses allocated to 'foo'. And, this was for an extremely simple scenario. Imagine dozens of programs and background services running at the same time and their memory locations being scattered all around leading to untraceable complexity.
Virtual Memory was introduced to overcome this issue. When an Operating System is using Virtual Memory, the programs can think they're being allocated consistent memory addresses starting from 0 and going forth. But behind the scenes, the OS might be allocating physical addresses from all over the place. This is achieved through a mapping between Physical and Virtual memory maintained by the Operating System.
Take a look at our example that uses Virtual Memory this time around:
Physical Memory Address | Virtual Memory Address | Allocated Program |
---|---|---|
0-999 | 0-999 | foo |
1000-1999 | 0-999 | bar |
2000-2999 | 1000-1999 | foo |
3000-3999 | - | - |
As is evident, 'foo' has been allocated—although virtual—a memory block containing addresses 0 through 1999. Yes, the physical addresses might be scattered but as far as 'foo' sees, it's been allocated a sequential block of memory to operate on.
File Access[edit]
A computer in the current day stores hundreds if not thousands of files in its Secondary memory. Hence, a few file access mechanisms were introduced for Operating Systems to adapt and swiftly handle I/O operations on their disk storage. Currently used File Access methods are threefold:
- Sequential Access
As the name suggests, in this mechanism, the file is accessed in a preset sequential order. This is the most basic and widely used method of the bunch.
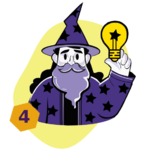
Try to imagine the functionality of a vintage magnetic audiotape of my age. Obviously, you can't skip here and there on it to read data from it, right?
So, much like a magnetic audiotape, the Sequential Access method performs file read and write operations in a sequential order.
- Random Access
As opposed to Sequential Access, Random Access method opts to access file records in a direct manner. This method directly places the file pointer on the required memory addresses and performs the required I/O operations.
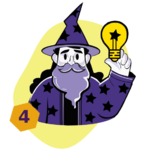
This is an instance where Random Access to files becomes useful.
- Indexed Sequential Access
Think of this method simply as a Sequential Access method performed on indexed files. When using this method the Operating System pre-indexes the file with unique keys given to each record. Whenever the Indexed Sequential Access Method wants to perform I/O operations on a record, it locates the required record with help of indexes.
User Interaction[edit]
In terms of User Interaction, Operating Systems have come a long way from the non-interactive batch processing systems used in the early days. Nowadays users interact with Operating Systems using a couple of methods:
- Command Line Interface (CLI)
This is a text-based interface where the user can give commands using an input method, e.g., keyboard, to command the OS to run processes and scripts. These commands can involve anything from traversing through the file hierarchy, performing read and write operations, executing I/O operations, etc.
- Graphical User Interface (GUI)
This is a graphical interface created to overcome the restrictions of a CLI. The user is presented with an interface containing windows, icons, menus, etc. Users can interact with the GUI using a plethora of input devices like a mouse, keyboard, joystick, microphone, or camera just to name a few. GUI gives way for a more user-friendly interaction with the OS.
Key Concepts[edit]
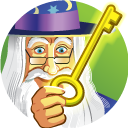
- Operating System acts as the sole mediator between the humans and the computer's hardware.
- OS makes a process traverse through many states. At any given time a started process could be in any one of these states: new, ready, running, waiting, terminated.
- Process Scheduling is when the OS analyzes and determines which processes to be moved to running state and which of them to be kept in the ready state.
- Process scheduling can be categorized as preemptive or non-preemptive scheduling based on how the currently running process is chosen.
- OS keeps track of memory locations on whether memory locations are currently allocated or free. Then it determines how much and when to allocate free memory to programs.
- Virtual Memory allocation avoids non-sequential blocks of memory being allocated to programs.
- Files on Operating Systems are accessed using Sequential Access, Random Access, or Indexed Sequential Access method.
- Users interact with the Operating System through a Command Line Interface(CLI) or a Graphical User Interface(GUI).