Pygame In Coder Merlin
How to Run Pygame in Coder Merlin[edit]
Prerequisites[edit]
- Learn the basics of Python
- Basics of Linux Commands (most important)
- Learn the basics of Pygame
- Learn how to use Google
Good Things to Know[edit]
- How to use VaporShell (outlined below)
- How to use Pygbag (how we are going to display in the Web, outlined below)
Steps[edit]
Step One: Prepare[edit]
Have your game/project ready. Know that the main loop has to be asynchronous to work so that Pygbag can work properly.
Here's an example of a basic Pygbag project from the Pygbag pypi
import asyncio
# Try to declare all your globals at once to facilitate compilation later.
COUNT_DOWN = 3
# Do init here
# Load any assets right now to avoid lag at runtime or network errors.
async def main():
global COUNT_DOWN
# avoid this kind declaration, prefer the way above
COUNT_DOWN = 3
while True:
# Do your rendering here, note that it's NOT an infinite loop,
# and it is fired only when VSYNC occurs
# Usually 1/60 or more times per seconds on desktop
# could be less on some mobile devices
print(f"""
Hello[{COUNT_DOWN}] from Python
""")
# pygame.display.update() should go right next line
await asyncio.sleep(0) # Very important, and keep it 0
if not COUNT_DOWN:
return
COUNT_DOWN = COUNT_DOWN - 1
# This is the program entry point:
asyncio.run(main())
# Do not add anything from here, especially sys.exit/pygame.quit
# asyncio.run is non-blocking on pygame-wasm and code would be executed
# right before program start main()
Some important things to note are that main()
is asynchronous and asyncio.sleep(0)
is used. This is used so that the function "gives up control" and can run other things like the Web page. For more information, see the Why does asyncio.sleep(0) make my code faster? page on Stackoverflow.
Step Two: Get Vapor Ready[edit]
We are using Vapor Client because it allows for more than static HTML and bare javascript. Therefore, we need to download it.
First, git clone VaporClient.
nikola-tesla@codermerlin:~$ git clone https://github.com/TheCoderMerlin/VaporShell
Then build and run it:
nikola-tesla@codermerlin:~/VaporShell$ build
nikola-tesla@codermerlin:~/VaporShell$ run
The server will then answer at https://www.codermerlin.com/vapor/(user-name)/.
(The above is from the Vapor Wiki)
After running it, note the port it says it is running on. This is important because any website you run on that port will load in VaporClient on the "answer" URL. Now that you have VaporClient ready, let's move on to step three!
Step Three: Install Pygbag[edit]
You can do this via:
pip install pygbag
Step Four: Run Pygbag[edit]
Run the below in the directory of your game/project.
python -m pygbag --archive .
Note: If python is not found, it might be labeled as "python3"
It creates the folder Build that contains a zip of your project in web form. This zip should be called web.zip.
This is not too important though because that build will already be unzipped in the web directory. cd web
into that directory and then run the web server with:
python -m http.server [port_number_here] &
Example:
python -m http.server 11937 &
This allows us to display the game at the vapor URL: https://www.codermerlin.academy/vapor/firstname-lastname/
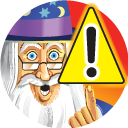
Make sure to have the slash at the end of the URL.
The &
allows us to run the server in the background. It is not required but it is good if you want to work on other things while the server is running. To stop the server from running do:
jobs
And note which job number the server is. Then do:
kill %(job number)
If it gives an error similar to:
OSError: [Errno 98] Address already in use
This means you already have a job running at that address. You need to kill it like killing the server.